Loading...
Searching...
No Matches
Public Member Functions |
Static Public Member Functions |
Protected Member Functions |
Protected Attributes |
List of all members
cv::rgbd::RgbdOdometry Class Reference
#include <opencv2/rgbd/depth.hpp>
Inheritance diagram for cv::rgbd::RgbdOdometry:
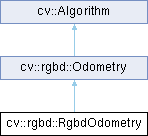
Public Member Functions | |
RgbdOdometry () | |
RgbdOdometry (const Mat &cameraMatrix, float minDepth=Odometry::DEFAULT_MIN_DEPTH(), float maxDepth=Odometry::DEFAULT_MAX_DEPTH(), float maxDepthDiff=Odometry::DEFAULT_MAX_DEPTH_DIFF(), const std::vector< int > &iterCounts=std::vector< int >(), const std::vector< float > &minGradientMagnitudes=std::vector< float >(), float maxPointsPart=Odometry::DEFAULT_MAX_POINTS_PART(), int transformType=Odometry::RIGID_BODY_MOTION) | |
cv::Mat | getCameraMatrix () const CV_OVERRIDE |
cv::Mat | getIterationCounts () const |
double | getMaxDepth () const |
double | getMaxDepthDiff () const |
double | getMaxPointsPart () const |
double | getMaxRotation () const |
double | getMaxTranslation () const |
double | getMinDepth () const |
cv::Mat | getMinGradientMagnitudes () const |
int | getTransformType () const CV_OVERRIDE |
virtual Size | prepareFrameCache (Ptr< OdometryFrame > &frame, int cacheType) const CV_OVERRIDE |
void | setCameraMatrix (const cv::Mat &val) CV_OVERRIDE |
void | setIterationCounts (const cv::Mat &val) |
void | setMaxDepth (double val) |
void | setMaxDepthDiff (double val) |
void | setMaxPointsPart (double val) |
void | setMaxRotation (double val) |
void | setMaxTranslation (double val) |
void | setMinDepth (double val) |
void | setMinGradientMagnitudes (const cv::Mat &val) |
void | setTransformType (int val) CV_OVERRIDE |
![]() | |
bool | compute (const Mat &srcImage, const Mat &srcDepth, const Mat &srcMask, const Mat &dstImage, const Mat &dstDepth, const Mat &dstMask, OutputArray Rt, const Mat &initRt=Mat()) const |
bool | compute (Ptr< OdometryFrame > &srcFrame, Ptr< OdometryFrame > &dstFrame, OutputArray Rt, const Mat &initRt=Mat()) const |
virtual cv::Mat | getCameraMatrix () const =0 |
virtual int | getTransformType () const =0 |
virtual Size | prepareFrameCache (Ptr< OdometryFrame > &frame, int cacheType) const |
virtual void | setCameraMatrix (const cv::Mat &val)=0 |
virtual void | setTransformType (int val)=0 |
![]() | |
Algorithm () | |
virtual | ~Algorithm () |
virtual void | clear () |
Clears the algorithm state. | |
virtual bool | empty () const |
Returns true if the Algorithm is empty (e.g. in the very beginning or after unsuccessful read. | |
virtual String | getDefaultName () const |
virtual void | read (const FileNode &fn) |
Reads algorithm parameters from a file storage. | |
virtual void | save (const String &filename) const |
void | write (const Ptr< FileStorage > &fs, const String &name=String()) const |
virtual void | write (FileStorage &fs) const |
Stores algorithm parameters in a file storage. | |
void | write (FileStorage &fs, const String &name) const |
Static Public Member Functions | |
static Ptr< RgbdOdometry > | create (const Mat &cameraMatrix=Mat(), float minDepth=Odometry::DEFAULT_MIN_DEPTH(), float maxDepth=Odometry::DEFAULT_MAX_DEPTH(), float maxDepthDiff=Odometry::DEFAULT_MAX_DEPTH_DIFF(), const std::vector< int > &iterCounts=std::vector< int >(), const std::vector< float > &minGradientMagnitudes=std::vector< float >(), float maxPointsPart=Odometry::DEFAULT_MAX_POINTS_PART(), int transformType=Odometry::RIGID_BODY_MOTION) |
![]() | |
static Ptr< Odometry > | create (const String &odometryType) |
static float | DEFAULT_MAX_DEPTH () |
static float | DEFAULT_MAX_DEPTH_DIFF () |
static float | DEFAULT_MAX_POINTS_PART () |
static float | DEFAULT_MAX_ROTATION () |
static float | DEFAULT_MAX_TRANSLATION () |
static float | DEFAULT_MIN_DEPTH () |
![]() | |
template<typename _Tp > | |
static Ptr< _Tp > | load (const String &filename, const String &objname=String()) |
Loads algorithm from the file. | |
template<typename _Tp > | |
static Ptr< _Tp > | loadFromString (const String &strModel, const String &objname=String()) |
Loads algorithm from a String. | |
template<typename _Tp > | |
static Ptr< _Tp > | read (const FileNode &fn) |
Reads algorithm from the file node. | |
Protected Member Functions | |
virtual void | checkParams () const CV_OVERRIDE |
virtual bool | computeImpl (const Ptr< OdometryFrame > &srcFrame, const Ptr< OdometryFrame > &dstFrame, OutputArray Rt, const Mat &initRt) const CV_OVERRIDE |
virtual void | checkParams () const =0 |
virtual bool | computeImpl (const Ptr< OdometryFrame > &srcFrame, const Ptr< OdometryFrame > &dstFrame, OutputArray Rt, const Mat &initRt) const =0 |
![]() | |
void | writeFormat (FileStorage &fs) const |
Protected Attributes | |
Mat | cameraMatrix |
Mat | iterCounts |
double | maxDepth |
double | maxDepthDiff |
double | maxPointsPart |
double | maxRotation |
double | maxTranslation |
double | minDepth |
Mat | minGradientMagnitudes |
int | transformType |
Additional Inherited Members | |
![]() | |
enum | { ROTATION = 1 , TRANSLATION = 2 , RIGID_BODY_MOTION = 4 } |
Detailed Description
Odometry based on the paper "Real-Time Visual Odometry from Dense RGB-D Images", F. Steinbucker, J. Strum, D. Cremers, ICCV, 2011.
Constructor & Destructor Documentation
◆ RgbdOdometry() [1/2]
cv::rgbd::RgbdOdometry::RgbdOdometry | ( | ) |
◆ RgbdOdometry() [2/2]
cv::rgbd::RgbdOdometry::RgbdOdometry | ( | const Mat & | cameraMatrix, |
float | minDepth = Odometry::DEFAULT_MIN_DEPTH() , |
||
float | maxDepth = Odometry::DEFAULT_MAX_DEPTH() , |
||
float | maxDepthDiff = Odometry::DEFAULT_MAX_DEPTH_DIFF() , |
||
const std::vector< int > & | iterCounts = std::vector< int >() , |
||
const std::vector< float > & | minGradientMagnitudes = std::vector< float >() , |
||
float | maxPointsPart = Odometry::DEFAULT_MAX_POINTS_PART() , |
||
int | transformType = Odometry::RIGID_BODY_MOTION |
||
) |
Constructor.
- Parameters
-
cameraMatrix Camera matrix minDepth Pixels with depth less than minDepth will not be used (in meters) maxDepth Pixels with depth larger than maxDepth will not be used (in meters) maxDepthDiff Correspondences between pixels of two given frames will be filtered out if their depth difference is larger than maxDepthDiff (in meters) iterCounts Count of iterations on each pyramid level. minGradientMagnitudes For each pyramid level the pixels will be filtered out if they have gradient magnitude less than minGradientMagnitudes[level]. maxPointsPart The method uses a random pixels subset of size frameWidth x frameHeight x pointsPart transformType Class of transformation
Member Function Documentation
◆ checkParams()
|
protectedvirtual |
Implements cv::rgbd::Odometry.
◆ computeImpl()
|
protectedvirtual |
Implements cv::rgbd::Odometry.
◆ create()
|
static |
◆ getCameraMatrix()
|
inlinevirtual |
- See also
- setCameraMatrix
Implements cv::rgbd::Odometry.
◆ getIterationCounts()
|
inline |
◆ getMaxDepth()
|
inline |
◆ getMaxDepthDiff()
|
inline |
◆ getMaxPointsPart()
|
inline |
◆ getMaxRotation()
|
inline |
◆ getMaxTranslation()
|
inline |
◆ getMinDepth()
|
inline |
◆ getMinGradientMagnitudes()
|
inline |
◆ getTransformType()
|
inlinevirtual |
- See also
- setTransformType
Implements cv::rgbd::Odometry.
◆ prepareFrameCache()
|
virtual |
Prepare a cache for the frame. The function checks the precomputed/passed data (throws the error if this data does not satisfy) and computes all remaining cache data needed for the frame. Returned size is a resolution of the prepared frame.
- Parameters
-
frame The odometry which will process the frame. cacheType The cache type: CACHE_SRC, CACHE_DST or CACHE_ALL.
Reimplemented from cv::rgbd::Odometry.
◆ setCameraMatrix()
|
inlinevirtual |
- See also
- getCameraMatrix
Implements cv::rgbd::Odometry.
◆ setIterationCounts()
|
inline |
◆ setMaxDepth()
|
inline |
◆ setMaxDepthDiff()
|
inline |
◆ setMaxPointsPart()
|
inline |
◆ setMaxRotation()
|
inline |
◆ setMaxTranslation()
|
inline |
◆ setMinDepth()
|
inline |
◆ setMinGradientMagnitudes()
|
inline |
◆ setTransformType()
|
inlinevirtual |
- See also
- getTransformType
Implements cv::rgbd::Odometry.
Member Data Documentation
◆ cameraMatrix
|
protected |
◆ iterCounts
|
protected |
◆ maxDepth
|
protected |
◆ maxDepthDiff
|
protected |
◆ maxPointsPart
|
protected |
◆ maxRotation
|
protected |
◆ maxTranslation
|
protected |
◆ minDepth
|
protected |
◆ minGradientMagnitudes
|
protected |
◆ transformType
|
protected |
The documentation for this class was generated from the following file:
- opencv2/rgbd/depth.hpp