Loading...
Searching...
No Matches
Public Types |
Public Member Functions |
Static Public Member Functions |
Protected Member Functions |
Protected Attributes |
List of all members
cv::rgbd::RgbdNormals Class Reference
#include <opencv2/rgbd/depth.hpp>
Inheritance diagram for cv::rgbd::RgbdNormals:
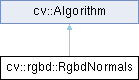
Public Types | |
enum | RGBD_NORMALS_METHOD { RGBD_NORMALS_METHOD_FALS = 0 , RGBD_NORMALS_METHOD_LINEMOD = 1 , RGBD_NORMALS_METHOD_SRI = 2 } |
Public Member Functions | |
RgbdNormals () | |
RgbdNormals (int rows, int cols, int depth, InputArray K, int window_size=5, int method=RgbdNormals::RGBD_NORMALS_METHOD_FALS) | |
~RgbdNormals () | |
int | getCols () const |
int | getDepth () const |
cv::Mat | getK () const |
int | getMethod () const |
int | getRows () const |
int | getWindowSize () const |
void | initialize () const |
void | operator() (InputArray points, OutputArray normals) const |
void | setCols (int val) |
void | setDepth (int val) |
void | setK (const cv::Mat &val) |
void | setMethod (int val) |
void | setRows (int val) |
void | setWindowSize (int val) |
![]() | |
Algorithm () | |
virtual | ~Algorithm () |
virtual void | clear () |
Clears the algorithm state. | |
virtual bool | empty () const |
Returns true if the Algorithm is empty (e.g. in the very beginning or after unsuccessful read. | |
virtual String | getDefaultName () const |
virtual void | read (const FileNode &fn) |
Reads algorithm parameters from a file storage. | |
virtual void | save (const String &filename) const |
void | write (const Ptr< FileStorage > &fs, const String &name=String()) const |
virtual void | write (FileStorage &fs) const |
Stores algorithm parameters in a file storage. | |
void | write (FileStorage &fs, const String &name) const |
Static Public Member Functions | |
static Ptr< RgbdNormals > | create (int rows, int cols, int depth, InputArray K, int window_size=5, int method=RgbdNormals::RGBD_NORMALS_METHOD_FALS) |
![]() | |
template<typename _Tp > | |
static Ptr< _Tp > | load (const String &filename, const String &objname=String()) |
Loads algorithm from the file. | |
template<typename _Tp > | |
static Ptr< _Tp > | loadFromString (const String &strModel, const String &objname=String()) |
Loads algorithm from a String. | |
template<typename _Tp > | |
static Ptr< _Tp > | read (const FileNode &fn) |
Reads algorithm from the file node. | |
Protected Member Functions | |
void | initialize_normals_impl (int rows, int cols, int depth, const Mat &K, int window_size, int method) const |
![]() | |
void | writeFormat (FileStorage &fs) const |
Protected Attributes | |
int | cols_ |
int | depth_ |
Mat | K_ |
int | method_ |
void * | rgbd_normals_impl_ |
int | rows_ |
int | window_size_ |
Detailed Description
Object that can compute the normals in an image. It is an object as it can cache data for speed efficiency The implemented methods are either:
- FALS (the fastest) and SRI from
Fast and Accurate Computation of Surface Normals from Range Images
by H. Badino, D. Huber, Y. Park and T. Kanade - the normals with bilateral filtering on a depth image from
Gradient Response Maps for Real-Time Detection of Texture-Less Objects
by S. Hinterstoisser, C. Cagniart, S. Ilic, P. Sturm, N. Navab, P. Fua, and V. Lepetit
Member Enumeration Documentation
◆ RGBD_NORMALS_METHOD
Constructor & Destructor Documentation
◆ RgbdNormals() [1/2]
|
inline |
◆ RgbdNormals() [2/2]
cv::rgbd::RgbdNormals::RgbdNormals | ( | int | rows, |
int | cols, | ||
int | depth, | ||
InputArray | K, | ||
int | window_size = 5 , |
||
int | method = RgbdNormals::RGBD_NORMALS_METHOD_FALS |
||
) |
Constructor
- Parameters
-
rows the number of rows of the depth image normals will be computed on cols the number of cols of the depth image normals will be computed on depth the depth of the normals (only CV_32F or CV_64F) K the calibration matrix to use window_size the window size to compute the normals: can only be 1,3,5 or 7 method one of the methods to use: RGBD_NORMALS_METHOD_SRI, RGBD_NORMALS_METHOD_FALS
◆ ~RgbdNormals()
cv::rgbd::RgbdNormals::~RgbdNormals | ( | ) |
Member Function Documentation
◆ create()
|
static |
◆ getCols()
|
inline |
◆ getDepth()
|
inline |
◆ getK()
|
inline |
◆ getMethod()
|
inline |
◆ getRows()
|
inline |
◆ getWindowSize()
|
inline |
◆ initialize()
void cv::rgbd::RgbdNormals::initialize | ( | ) | const |
Initializes some data that is cached for later computation If that function is not called, it will be called the first time normals are computed
◆ initialize_normals_impl()
|
protected |
◆ operator()()
void cv::rgbd::RgbdNormals::operator() | ( | InputArray | points, |
OutputArray | normals | ||
) | const |
Given a set of 3d points in a depth image, compute the normals at each point.
- Parameters
-
points a rows x cols x 3 matrix of CV_32F/CV64F or a rows x cols x 1 CV_U16S normals a rows x cols x 3 matrix
◆ setCols()
|
inline |
◆ setDepth()
|
inline |
◆ setK()
|
inline |
◆ setMethod()
|
inline |
◆ setRows()
|
inline |
◆ setWindowSize()
|
inline |
Member Data Documentation
◆ cols_
|
protected |
◆ depth_
|
protected |
◆ K_
|
protected |
◆ method_
|
protected |
◆ rgbd_normals_impl_
|
mutableprotected |
◆ rows_
|
protected |
◆ window_size_
|
protected |
The documentation for this class was generated from the following file:
- opencv2/rgbd/depth.hpp