Loading...
Searching...
No Matches
cv::rgbd::RgbdPlane Class Reference
#include <opencv2/rgbd/depth.hpp>
Inheritance diagram for cv::rgbd::RgbdPlane:
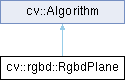
Public Types | |
enum | RGBD_PLANE_METHOD { RGBD_PLANE_METHOD_DEFAULT } |
Public Member Functions | |
RgbdPlane (int method, int block_size, int min_size, double threshold, double sensor_error_a=0, double sensor_error_b=0, double sensor_error_c=0) | |
RgbdPlane (int method=RgbdPlane::RGBD_PLANE_METHOD_DEFAULT) | |
~RgbdPlane () | |
int | getBlockSize () const |
int | getMethod () const |
int | getMinSize () const |
double | getSensorErrorA () const |
double | getSensorErrorB () const |
double | getSensorErrorC () const |
double | getThreshold () const |
void | operator() (InputArray points3d, InputArray normals, OutputArray mask, OutputArray plane_coefficients) |
void | operator() (InputArray points3d, OutputArray mask, OutputArray plane_coefficients) |
void | setBlockSize (int val) |
void | setMethod (int val) |
void | setMinSize (int val) |
void | setSensorErrorA (double val) |
void | setSensorErrorB (double val) |
void | setSensorErrorC (double val) |
void | setThreshold (double val) |
![]() | |
Algorithm () | |
virtual | ~Algorithm () |
virtual void | clear () |
Clears the algorithm state. | |
virtual bool | empty () const |
Returns true if the Algorithm is empty (e.g. in the very beginning or after unsuccessful read. | |
virtual String | getDefaultName () const |
virtual void | read (const FileNode &fn) |
Reads algorithm parameters from a file storage. | |
virtual void | save (const String &filename) const |
void | write (const Ptr< FileStorage > &fs, const String &name=String()) const |
virtual void | write (FileStorage &fs) const |
Stores algorithm parameters in a file storage. | |
void | write (FileStorage &fs, const String &name) const |
Static Public Member Functions | |
static Ptr< RgbdPlane > | create (int method, int block_size, int min_size, double threshold, double sensor_error_a=0, double sensor_error_b=0, double sensor_error_c=0) |
![]() | |
template<typename _Tp > | |
static Ptr< _Tp > | load (const String &filename, const String &objname=String()) |
Loads algorithm from the file. | |
template<typename _Tp > | |
static Ptr< _Tp > | loadFromString (const String &strModel, const String &objname=String()) |
Loads algorithm from a String. | |
template<typename _Tp > | |
static Ptr< _Tp > | read (const FileNode &fn) |
Reads algorithm from the file node. | |
Additional Inherited Members | |
![]() | |
void | writeFormat (FileStorage &fs) const |
Detailed Description
Object that can compute planes in an image
Member Enumeration Documentation
◆ RGBD_PLANE_METHOD
Constructor & Destructor Documentation
◆ RgbdPlane() [1/2]
|
inline |
◆ RgbdPlane() [2/2]
cv::rgbd::RgbdPlane::RgbdPlane | ( | int | method, |
int | block_size, | ||
int | min_size, | ||
double | threshold, | ||
double | sensor_error_a = 0 , |
||
double | sensor_error_b = 0 , |
||
double | sensor_error_c = 0 |
||
) |
Constructor
- Parameters
-
block_size The size of the blocks to look at for a stable MSE min_size The minimum size of a cluster to be considered a plane threshold The maximum distance of a point from a plane to belong to it (in meters) sensor_error_a coefficient of the sensor error. 0 by default, 0.0075 for a Kinect sensor_error_b coefficient of the sensor error. 0 by default sensor_error_c coefficient of the sensor error. 0 by default method The method to use to compute the planes.
◆ ~RgbdPlane()
cv::rgbd::RgbdPlane::~RgbdPlane | ( | ) |
Member Function Documentation
◆ create()
|
static |
◆ getBlockSize()
|
inline |
◆ getMethod()
|
inline |
◆ getMinSize()
|
inline |
◆ getSensorErrorA()
|
inline |
◆ getSensorErrorB()
|
inline |
◆ getSensorErrorC()
|
inline |
◆ getThreshold()
|
inline |
◆ operator()() [1/2]
void cv::rgbd::RgbdPlane::operator() | ( | InputArray | points3d, |
InputArray | normals, | ||
OutputArray | mask, | ||
OutputArray | plane_coefficients | ||
) |
Find The planes in a depth image
- Parameters
-
points3d the 3d points organized like the depth image: rows x cols with 3 channels normals the normals for every point in the depth image mask An image where each pixel is labeled with the plane it belongs to and 255 if it does not belong to any plane plane_coefficients the coefficients of the corresponding planes (a,b,c,d) such that ax+by+cz+d=0, norm(a,b,c)=1 and c < 0 (so that the normal points towards the camera)
◆ operator()() [2/2]
void cv::rgbd::RgbdPlane::operator() | ( | InputArray | points3d, |
OutputArray | mask, | ||
OutputArray | plane_coefficients | ||
) |
Find The planes in a depth image but without doing a normal check, which is faster but less accurate
- Parameters
-
points3d the 3d points organized like the depth image: rows x cols with 3 channels mask An image where each pixel is labeled with the plane it belongs to and 255 if it does not belong to any plane plane_coefficients the coefficients of the corresponding planes (a,b,c,d) such that ax+by+cz+d=0
◆ setBlockSize()
|
inline |
◆ setMethod()
|
inline |
◆ setMinSize()
|
inline |
◆ setSensorErrorA()
|
inline |
◆ setSensorErrorB()
|
inline |
◆ setSensorErrorC()
|
inline |
◆ setThreshold()
|
inline |
The documentation for this class was generated from the following file:
- opencv2/rgbd/depth.hpp