Class implementing DAISY descriptor, described in [Tola10]. More...
#include <opencv2/xfeatures2d.hpp>
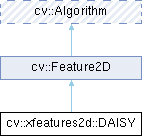
Public Types | |
enum | NormalizationType { NRM_NONE = 100 , NRM_PARTIAL = 101 , NRM_FULL = 102 , NRM_SIFT = 103 } |
Public Member Functions | |
virtual void | compute (InputArray image, OutputArray descriptors)=0 |
virtual void | compute (InputArray image, Rect roi, OutputArray descriptors)=0 |
virtual void | compute (InputArray image, std::vector< KeyPoint > &keypoints, OutputArray descriptors) CV_OVERRIDE=0 |
virtual void | compute (InputArrayOfArrays images, std::vector< std::vector< KeyPoint > > &keypoints, OutputArrayOfArrays descriptors) CV_OVERRIDE |
String | getDefaultName () const CV_OVERRIDE |
virtual void | GetDescriptor (double y, double x, int orientation, float *descriptor) const =0 |
virtual bool | GetDescriptor (double y, double x, int orientation, float *descriptor, double *H) const =0 |
virtual cv::Mat | getH () const =0 |
virtual bool | getInterpolation () const =0 |
virtual int | getNorm () const =0 |
virtual int | getQHist () const =0 |
virtual int | getQRadius () const =0 |
virtual int | getQTheta () const =0 |
virtual float | getRadius () const =0 |
virtual void | GetUnnormalizedDescriptor (double y, double x, int orientation, float *descriptor) const =0 |
virtual bool | GetUnnormalizedDescriptor (double y, double x, int orientation, float *descriptor, double *H) const =0 |
virtual bool | getUseOrientation () const =0 |
virtual void | setH (InputArray H)=0 |
virtual void | setInterpolation (bool interpolation)=0 |
virtual void | setNorm (int norm)=0 |
virtual void | setQHist (int q_hist)=0 |
virtual void | setQRadius (int q_radius)=0 |
virtual void | setQTheta (int q_theta)=0 |
virtual void | setRadius (float radius)=0 |
virtual void | setUseOrientation (bool use_orientation)=0 |
![]() | |
virtual | ~Feature2D () |
virtual void | compute (InputArray image, std::vector< KeyPoint > &keypoints, OutputArray descriptors) |
Computes the descriptors for a set of keypoints detected in an image (first variant) or image set (second variant). | |
virtual void | compute (InputArrayOfArrays images, std::vector< std::vector< KeyPoint > > &keypoints, OutputArrayOfArrays descriptors) |
virtual int | defaultNorm () const |
virtual int | descriptorSize () const |
virtual int | descriptorType () const |
virtual void | detect (InputArray image, std::vector< KeyPoint > &keypoints, InputArray mask=noArray()) |
Detects keypoints in an image (first variant) or image set (second variant). | |
virtual void | detect (InputArrayOfArrays images, std::vector< std::vector< KeyPoint > > &keypoints, InputArrayOfArrays masks=noArray()) |
virtual void | detectAndCompute (InputArray image, InputArray mask, std::vector< KeyPoint > &keypoints, OutputArray descriptors, bool useProvidedKeypoints=false) |
virtual bool | empty () const CV_OVERRIDE |
Return true if detector object is empty. | |
virtual String | getDefaultName () const CV_OVERRIDE |
virtual void | read (const FileNode &) CV_OVERRIDE |
Reads algorithm parameters from a file storage. | |
void | read (const String &fileName) |
void | write (const Ptr< FileStorage > &fs, const String &name) const |
void | write (const String &fileName) const |
virtual void | write (FileStorage &) const CV_OVERRIDE |
Stores algorithm parameters in a file storage. | |
void | write (FileStorage &fs, const String &name) const |
![]() | |
Algorithm () | |
virtual | ~Algorithm () |
virtual void | clear () |
Clears the algorithm state. | |
virtual bool | empty () const |
Returns true if the Algorithm is empty (e.g. in the very beginning or after unsuccessful read. | |
virtual String | getDefaultName () const |
virtual void | read (const FileNode &fn) |
Reads algorithm parameters from a file storage. | |
virtual void | save (const String &filename) const |
void | write (const Ptr< FileStorage > &fs, const String &name=String()) const |
virtual void | write (FileStorage &fs) const |
Stores algorithm parameters in a file storage. | |
void | write (FileStorage &fs, const String &name) const |
Static Public Member Functions | |
static Ptr< DAISY > | create (float radius=15, int q_radius=3, int q_theta=8, int q_hist=8, DAISY::NormalizationType norm=DAISY::NRM_NONE, InputArray H=noArray(), bool interpolation=true, bool use_orientation=false) |
![]() | |
template<typename _Tp > | |
static Ptr< _Tp > | load (const String &filename, const String &objname=String()) |
Loads algorithm from the file. | |
template<typename _Tp > | |
static Ptr< _Tp > | loadFromString (const String &strModel, const String &objname=String()) |
Loads algorithm from a String. | |
template<typename _Tp > | |
static Ptr< _Tp > | read (const FileNode &fn) |
Reads algorithm from the file node. | |
Additional Inherited Members | |
![]() | |
void | writeFormat (FileStorage &fs) const |
Detailed Description
Class implementing DAISY descriptor, described in [Tola10].
- Parameters
-
radius radius of the descriptor at the initial scale q_radius amount of radial range division quantity q_theta amount of angular range division quantity q_hist amount of gradient orientations range division quantity norm choose descriptors normalization type, where DAISY::NRM_NONE will not do any normalization (default), DAISY::NRM_PARTIAL mean that histograms are normalized independently for L2 norm equal to 1.0, DAISY::NRM_FULL mean that descriptors are normalized for L2 norm equal to 1.0, DAISY::NRM_SIFT mean that descriptors are normalized for L2 norm equal to 1.0 but no individual one is bigger than 0.154 as in SIFT H optional 3x3 homography matrix used to warp the grid of daisy but sampling keypoints remains unwarped on image interpolation switch to disable interpolation for speed improvement at minor quality loss use_orientation sample patterns using keypoints orientation, disabled by default.
Member Enumeration Documentation
◆ NormalizationType
Member Function Documentation
◆ compute() [1/4]
|
pure virtual |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
- Parameters
-
image image to extract descriptors descriptors resulted descriptors array for all image pixels
◆ compute() [2/4]
|
pure virtual |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
- Parameters
-
image image to extract descriptors roi region of interest within image descriptors resulted descriptors array for roi image pixels
◆ compute() [3/4]
|
pure virtual |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
- Parameters
-
image image to extract descriptors keypoints of interest within image descriptors resulted descriptors array
Reimplemented from cv::Feature2D.
◆ compute() [4/4]
|
virtual |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
- Parameters
-
images Image set. keypoints Input collection of keypoints. Keypoints for which a descriptor cannot be computed are removed. Sometimes new keypoints can be added, for example: SIFT duplicates keypoint with several dominant orientations (for each orientation). descriptors Computed descriptors. In the second variant of the method descriptors[i] are descriptors computed for a keypoints[i]. Row j is the keypoints (or keypoints[i]) is the descriptor for keypoint j-th keypoint.
Reimplemented from cv::Feature2D.
◆ create()
|
static |
◆ getDefaultName()
|
virtual |
Returns the algorithm string identifier. This string is used as top level xml/yml node tag when the object is saved to a file or string.
Reimplemented from cv::Feature2D.
◆ GetDescriptor() [1/2]
|
pure virtual |
- Parameters
-
y position y on image x position x on image orientation orientation on image (0->360) descriptor supplied array for descriptor storage
◆ GetDescriptor() [2/2]
|
pure virtual |
- Parameters
-
y position y on image x position x on image orientation orientation on image (0->360) descriptor supplied array for descriptor storage H homography matrix for warped grid
◆ getH()
|
pure virtual |
◆ getInterpolation()
|
pure virtual |
◆ getNorm()
|
pure virtual |
◆ getQHist()
|
pure virtual |
◆ getQRadius()
|
pure virtual |
◆ getQTheta()
|
pure virtual |
◆ getRadius()
|
pure virtual |
◆ GetUnnormalizedDescriptor() [1/2]
|
pure virtual |
- Parameters
-
y position y on image x position x on image orientation orientation on image (0->360) descriptor supplied array for descriptor storage
◆ GetUnnormalizedDescriptor() [2/2]
|
pure virtual |
- Parameters
-
y position y on image x position x on image orientation orientation on image (0->360) descriptor supplied array for descriptor storage H homography matrix for warped grid
◆ getUseOrientation()
|
pure virtual |
◆ setH()
|
pure virtual |
◆ setInterpolation()
|
pure virtual |
◆ setNorm()
|
pure virtual |
◆ setQHist()
|
pure virtual |
◆ setQRadius()
|
pure virtual |
◆ setQTheta()
|
pure virtual |
◆ setRadius()
|
pure virtual |
◆ setUseOrientation()
|
pure virtual |
The documentation for this class was generated from the following file:
- opencv2/xfeatures2d.hpp