Loading...
Searching...
No Matches
cv::ximgproc::FastLineDetector Class Referenceabstract
Class implementing the FLD (Fast Line Detector) algorithm described in [Lee14] . More...
#include <opencv2/ximgproc/fast_line_detector.hpp>
Inheritance diagram for cv::ximgproc::FastLineDetector:

Public Member Functions | |
virtual | ~FastLineDetector () |
virtual void | detect (InputArray image, OutputArray lines)=0 |
Finds lines in the input image. This is the output of the default parameters of the algorithm on the above shown image. | |
virtual void | drawSegments (InputOutputArray image, InputArray lines, bool draw_arrow=false, Scalar linecolor=Scalar(0, 0, 255), int linethickness=1)=0 |
Draws the line segments on a given image. | |
![]() | |
Algorithm () | |
virtual | ~Algorithm () |
virtual void | clear () |
Clears the algorithm state. | |
virtual bool | empty () const |
Returns true if the Algorithm is empty (e.g. in the very beginning or after unsuccessful read. | |
virtual String | getDefaultName () const |
virtual void | read (const FileNode &fn) |
Reads algorithm parameters from a file storage. | |
virtual void | save (const String &filename) const |
void | write (const Ptr< FileStorage > &fs, const String &name=String()) const |
virtual void | write (FileStorage &fs) const |
Stores algorithm parameters in a file storage. | |
void | write (FileStorage &fs, const String &name) const |
Additional Inherited Members | |
![]() | |
template<typename _Tp > | |
static Ptr< _Tp > | load (const String &filename, const String &objname=String()) |
Loads algorithm from the file. | |
template<typename _Tp > | |
static Ptr< _Tp > | loadFromString (const String &strModel, const String &objname=String()) |
Loads algorithm from a String. | |
template<typename _Tp > | |
static Ptr< _Tp > | read (const FileNode &fn) |
Reads algorithm from the file node. | |
![]() | |
void | writeFormat (FileStorage &fs) const |
Detailed Description
Class implementing the FLD (Fast Line Detector) algorithm described in [Lee14] .
#include <iostream>
#include "opencv2/imgproc.hpp"
#include "opencv2/ximgproc.hpp"
#include "opencv2/imgcodecs.hpp"
#include "opencv2/highgui.hpp"
using namespace std;
using namespace cv;
using namespace cv::ximgproc;
int main(int argc, char** argv)
{
string in;
CommandLineParser parser(argc, argv, "{@input|corridor.jpg|input image}{help h||show help message}");
if (parser.has("help"))
{
parser.printMessage();
return 0;
}
if( image.empty() )
{
return -1;
}
// Create FLD detector
// Param Default value Description
// length_threshold 10 - Segments shorter than this will be discarded
// distance_threshold 1.41421356 - A point placed from a hypothesis line
// segment farther than this will be
// regarded as an outlier
// canny_th1 50 - First threshold for
// hysteresis procedure in Canny()
// canny_th2 50 - Second threshold for
// hysteresis procedure in Canny()
// canny_aperture_size 3 - Aperturesize for the sobel operator in Canny().
// If zero, Canny() is not applied and the input
// image is taken as an edge image.
// do_merge false - If true, incremental merging of segments
// will be performed
int length_threshold = 10;
float distance_threshold = 1.41421356f;
double canny_th1 = 50.0;
double canny_th2 = 50.0;
int canny_aperture_size = 3;
bool do_merge = false;
Ptr<FastLineDetector> fld = createFastLineDetector(length_threshold,
distance_threshold, canny_th1, canny_th2, canny_aperture_size,
do_merge);
vector<Vec4f> lines;
// Because of some CPU's power strategy, it seems that the first running of
// an algorithm takes much longer. So here we run the algorithm 10 times
// to see the algorithm's processing time with sufficiently warmed-up
// CPU performance.
for (int run_count = 0; run_count < 5; run_count++) {
double freq = getTickFrequency();
lines.clear();
int64 start = getTickCount();
// Detect the lines with FLD
fld->detect(image, lines);
double duration_ms = double(getTickCount() - start) * 1000 / freq;
cout << "Elapsed time for FLD " << duration_ms << " ms." << endl;
}
// Show found lines with FLD
Mat line_image_fld(image);
fld->drawSegments(line_image_fld, lines);
imshow("FLD result", line_image_fld);
waitKey(1);
Ptr<EdgeDrawing> ed = createEdgeDrawing();
ed->params.EdgeDetectionOperator = EdgeDrawing::SOBEL;
ed->params.GradientThresholdValue = 38;
ed->params.AnchorThresholdValue = 8;
vector<Vec6d> ellipses;
for (int run_count = 0; run_count < 5; run_count++) {
double freq = getTickFrequency();
lines.clear();
int64 start = getTickCount();
// Detect edges
//you should call this before detectLines() and detectEllipses()
ed->detectEdges(image);
// Detect lines
ed->detectLines(lines);
double duration_ms = double(getTickCount() - start) * 1000 / freq;
cout << "Elapsed time for EdgeDrawing detectLines " << duration_ms << " ms." << endl;
start = getTickCount();
// Detect circles and ellipses
ed->detectEllipses(ellipses);
duration_ms = double(getTickCount() - start) * 1000 / freq;
cout << "Elapsed time for EdgeDrawing detectEllipses " << duration_ms << " ms." << endl;
}
vector<vector<Point> > segments = ed->getSegments();
for (size_t i = 0; i < segments.size(); i++)
{
const Point* pts = &segments[i][0];
int n = (int)segments[i].size();
polylines(edge_image_ed, &pts, &n, 1, false, Scalar((rand() & 255), (rand() & 255), (rand() & 255)), 1);
}
imshow("EdgeDrawing detected edges", edge_image_ed);
Mat line_image_ed(image);
fld->drawSegments(line_image_ed, lines);
// Draw circles and ellipses
for (size_t i = 0; i < ellipses.size(); i++)
{
double angle(ellipses[i][5]);
}
imshow("EdgeDrawing result", line_image_ed);
waitKey();
return 0;
}
static CV_NODISCARD_STD MatExpr zeros(int rows, int cols, int type)
Returns a zero array of the specified size and type.
cv::String findFile(const cv::String &relative_path, bool required=true, bool silentMode=false)
Try to find requested data file.
void imshow(const String &winname, InputArray mat)
Displays an image in the specified window.
@ IMREAD_GRAYSCALE
If set, always convert image to the single channel grayscale image (codec internal conversion).
Definition: imgcodecs.hpp:71
CV_EXPORTS_W Mat imread(const String &filename, int flags=IMREAD_COLOR)
Loads an image from a file.
void ellipse(InputOutputArray img, Point center, Size axes, double angle, double startAngle, double endAngle, const Scalar &color, int thickness=1, int lineType=LINE_8, int shift=0)
Draws a simple or thick elliptic arc or fills an ellipse sector.
void polylines(InputOutputArray img, InputArrayOfArrays pts, bool isClosed, const Scalar &color, int thickness=1, int lineType=LINE_8, int shift=0)
Draws several polygonal curves.
Ptr< EdgeDrawing > createEdgeDrawing()
Creates a smart pointer to a EdgeDrawing object and initializes it.
Ptr< FastLineDetector > createFastLineDetector(int length_threshold=10, float distance_threshold=1.414213562f, double canny_th1=50.0, double canny_th2=50.0, int canny_aperture_size=3, bool do_merge=false)
Creates a smart pointer to a FastLineDetector object and initializes it.
Definition: ximgproc.hpp:125
"black box" representation of the file storage associated with a file on disk.
Definition: core.hpp:106
STL namespace.
Constructor & Destructor Documentation
◆ ~FastLineDetector()
|
inlinevirtual |
Member Function Documentation
◆ detect()
|
pure virtual |
Finds lines in the input image. This is the output of the default parameters of the algorithm on the above shown image.
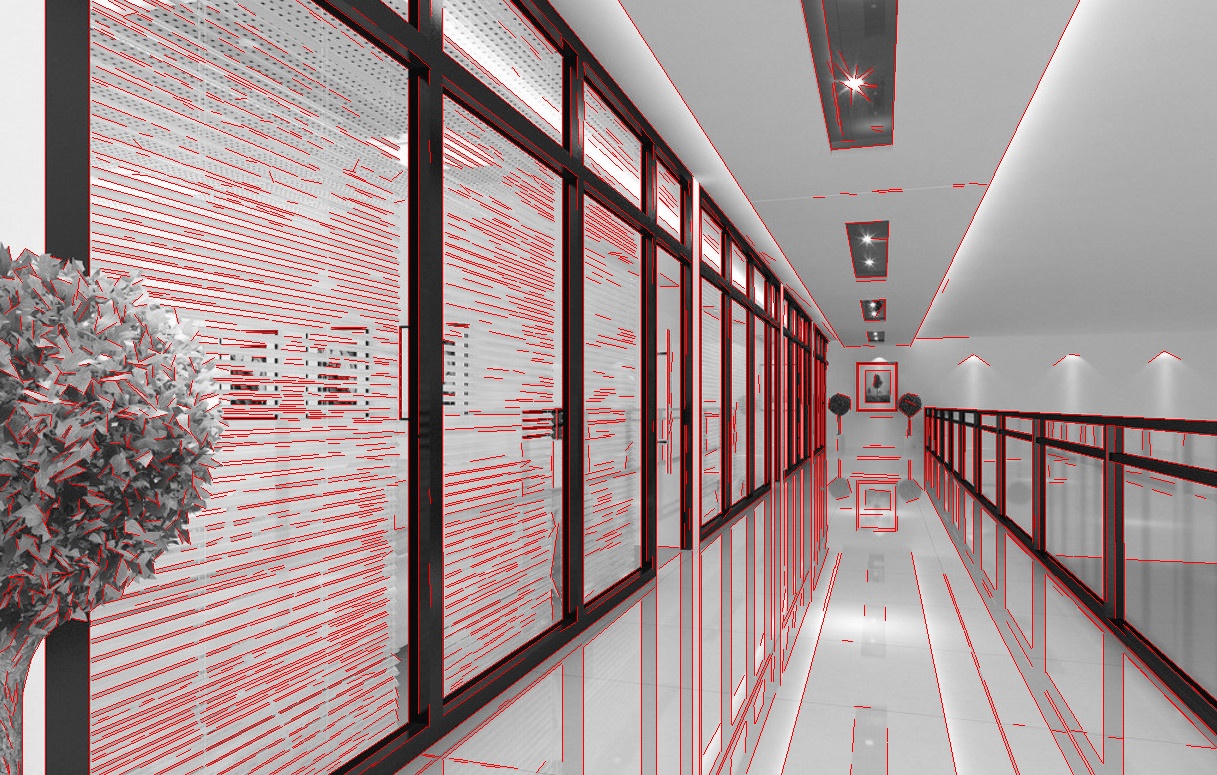
image
- Parameters
-
image A grayscale (CV_8UC1) input image. If only a roi needs to be selected, use: fld_ptr-\>detect(image(roi), lines, ...); lines += Scalar(roi.x, roi.y, roi.x, roi.y);
lines A vector of Vec4f elements specifying the beginning and ending point of a line. Where Vec4f is (x1, y1, x2, y2), point 1 is the start, point 2 - end. Returned lines are directed so that the brighter side is on their left.
◆ drawSegments()
|
pure virtual |
Draws the line segments on a given image.
- Parameters
-
image The image, where the lines will be drawn. Should be bigger or equal to the image, where the lines were found. lines A vector of the lines that needed to be drawn. draw_arrow If true, arrow heads will be drawn. linecolor Line color. linethickness Line thickness.
The documentation for this class was generated from the following file:
- opencv2/ximgproc/fast_line_detector.hpp